.NET MAUI Shell navigation is a URI-based navigation that uses routes to navigate to any page in the app. This is done without having to follow a set navigation hierarchy.
To register a route to navigate to a page called PageTwo.xaml, the code will look like this:
Routing.RegisterRoute("PageTwo", typeof(PageTwo));
However, my preferred method is to use the name of the page, so the code will follow this pattern to avoid magic strings flying around in the code
Routing.RegisterRoute(nameof(PageTwo), typeof(PageTwo));
THE PROBLEM
Now, consider having over fifty (50) pages in your application and you are using shell to handle the navigation. It means that you will have over fifty repeated lines of code to register the pages one after the other like this.
Routing.RegisterRoute(nameof(PageTwo), typeof(PageTwo));
Routing.RegisterRoute(nameof(PageThree), typeof(PageThree));
Routing.RegisterRoute(nameof(PageFour), typeof(PageFour));
//.......More Page.........
Routing.RegisterRoute(nameof(PageTwentyNine), typeof(PageTwentyNine));
Routing.RegisterRoute(nameof(PageThirty), typeof(PageThirty));
//.......More Page.........
Routing.RegisterRoute(nameof(PageFifty), typeof(PageFifty));
Routing.RegisterRoute(nameof(PageFiftyOne), typeof(PageFiftyOne));
There should be a better way, right?
THE SOLUTION
The solution contains just a few steps.
Step 1
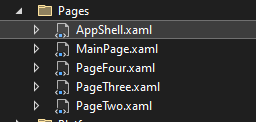
Ensure that the Shell (AppShell.xaml or whatever name you call it) is in the same folder (namespace) as the rest of the pages you want to register.
Step 2
Create an extension method in a class somewhere in your code (depending on how you structure your project) to scan for all the Types in that namespace and register each of them other than the shell that called the extension method.
public static void RegisterRoutes(this Shell shell)
{
var assembly = shell.GetType().Assembly;
var types = assembly.GetTypes().Where(t => string.Equals(t.Namespace, shell.GetType().Namespace, StringComparison.Ordinal)
&& t != shell.GetType()).ToArray();
foreach ( var type in types)
Routing.RegisterRoute(type.Name, type);
}
Step 3
In the constructor of the Shell (AppShell.xaml or whatever name you call it), call the extension method that you have just created.
public partial class AppShell : Shell
{
public AppShell()
{
InitializeComponent();
this.RegisterRoutes();
}
}
You can check out this video to see how this was implemented;